class: center, middle, inverse # Android ### Primera app .footnote[Mateu Yábar] --- # Objectiu final .fullscreen70[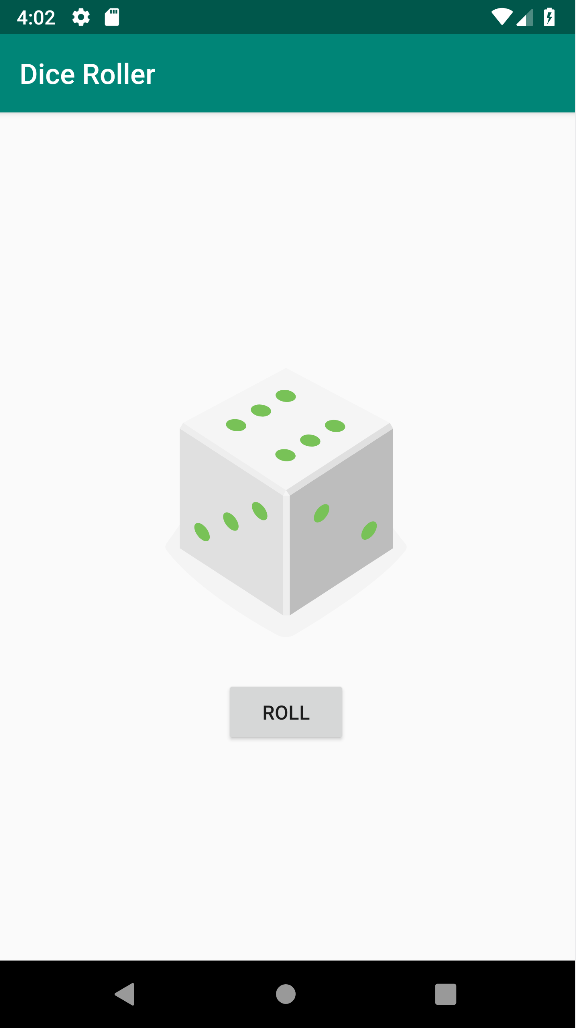] --- # Objectiu inicial .fullscreen70[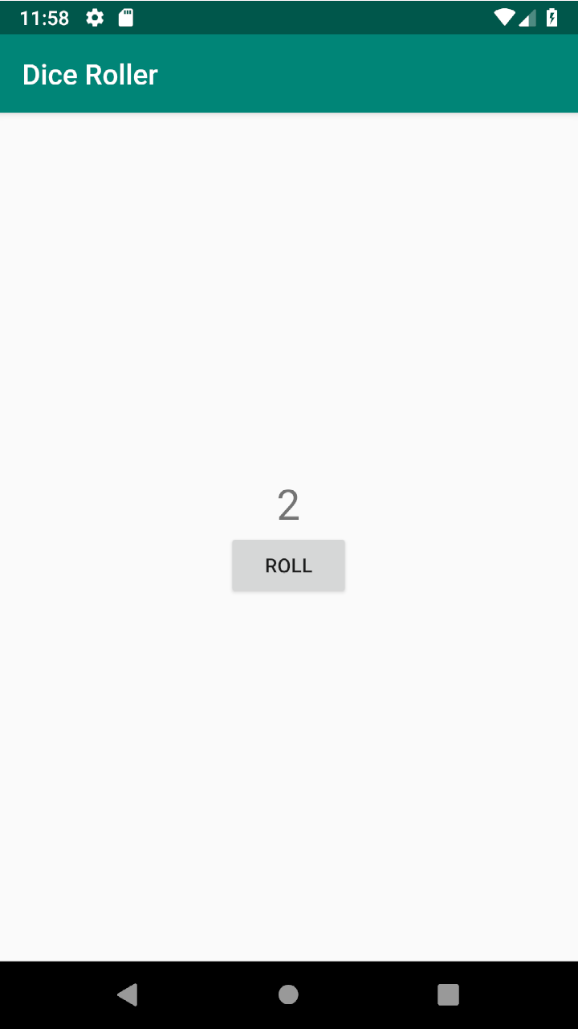] --- # Programació Android - Entorn - Android Studio - Llenguatges de programació - Java / Kotlin - Xml - Layouts - Strings - Styles - Colors - Navegació - (...) --- # Activity - Una Activity és un component de l'aplicació que conté una pantalla amb la qual els usuaris poden interactuar per realitzar una acció, com marcar un número de telèfon, fer una foto, enviar un correu electrònic o veure un mapa. - Una aplicació generalment consisteix en múltiples activitats vinculades de manera flexible entre si. --- # Explicació en viu - Creació d'un projecte amb una Activity a l'Android Studio. - Visió del codi - Java - Xml (grafix i text) - Manifest - Gradle - Execució --- # Exercici - Creeu un projecte Android, mireu els fitxes i executeu l'aplicació en un emulador. - L'aplicació s'ha de dir _cat.itb.diceroller_
--- # View - ViewGroup - Layout - Una __View__ generalment __dibuixa alguna cosa__ amb què l'usuari pot veure i interactuar. - Mentre que un __ViewGroup__ és un __contenidor__ invisible que defineix l'estructura de disseny per a Views i altres objectes ViewGroup - Tots els elements del disseny es creen mitjançant una jerarquia d'objectes View i ViewGroup. - Un __Layout__ defineix l'__estructura__ d'una interfície d'usuari de l'aplicació, com per exemple en una Activity. --- # Views .fullscreen70[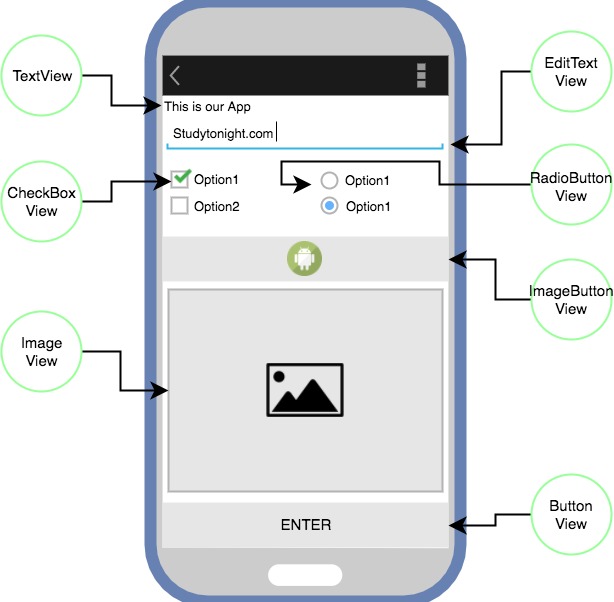] --- # Layout .fullscreen70[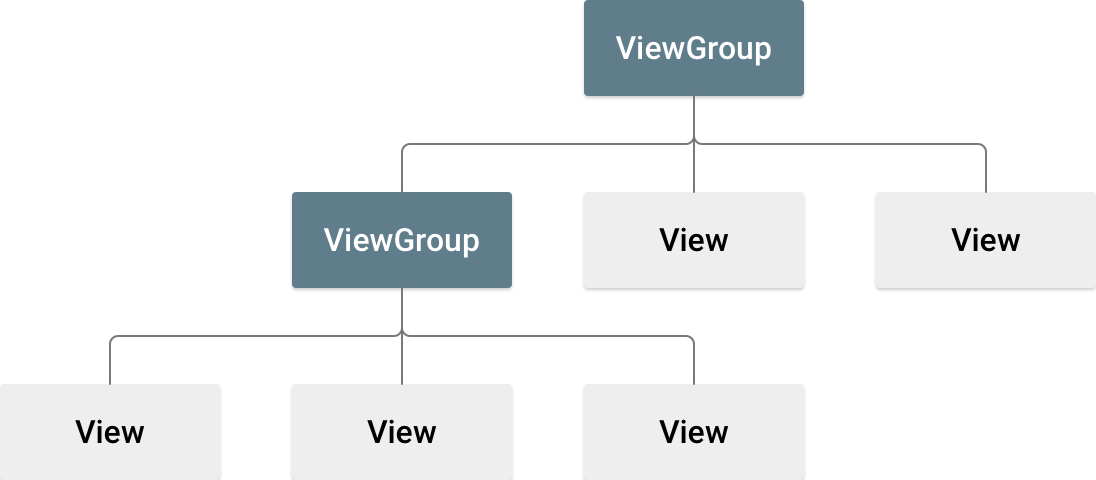] --- # ViewGroups - CoordinatorLayout - FrameLayout - LinearLayout - RelativeLayout - Toolbar --- # LinearLayout .near_full_width[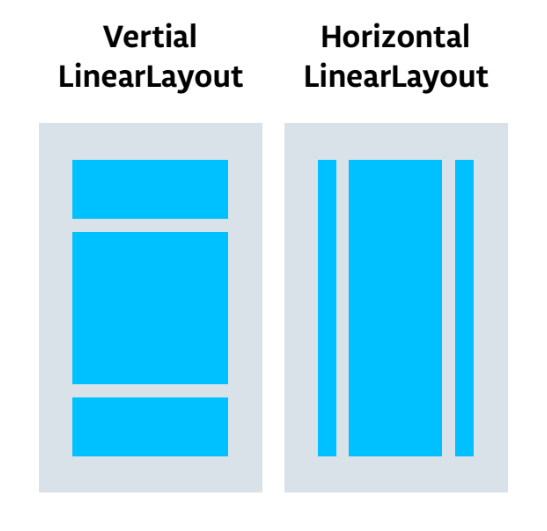] --- # LinearLayout - en viu - LinearLayout - orientation - views filles - layout_height / layout_width - layout_weight - layout_gravity --- # LinearLayout sample ```xml
``` --- # TextView - en viu - Mostra un text - Algunes propietats d'un TextView - id - text - textSize - sp Scale-independent Pixels - this is like the dp unit, but it is also scaled by the user's font size preference. It is recommended you use this unit when specifying font sizes, so they will be adjusted for both the screen density and user's preference. --- # Button - en viu - Mostra un botó amb un text - Algunes propietats d'un Button - id - text --- # String Resources - strings.xml - tools:text --- .fullscreen70[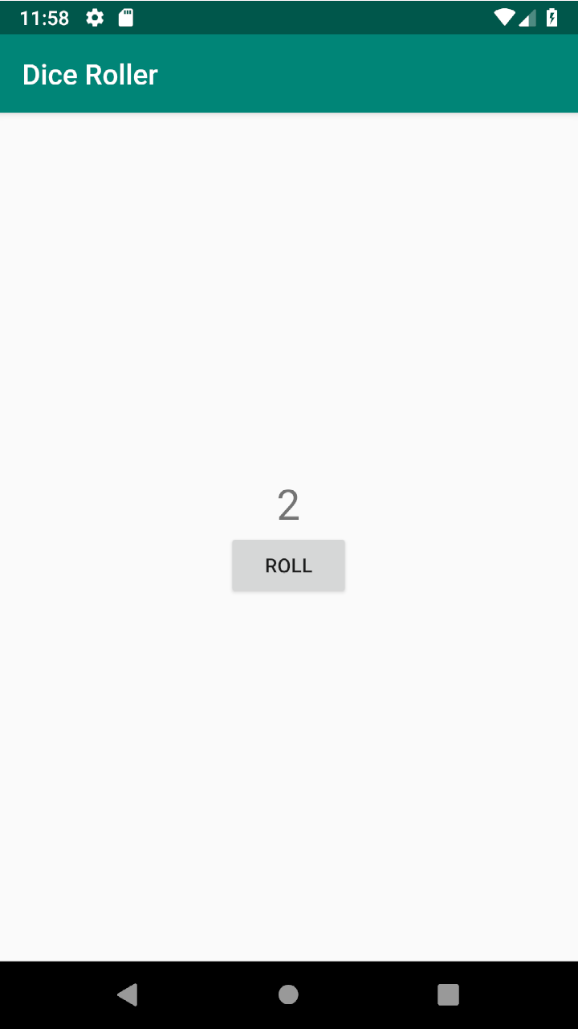] .footnote[
] --- # Enable Java 8 Afegir al gradle ```groovy android { ... compileOptions { sourceCompatibility JavaVersion.VERSION_1_8 targetCompatibility JavaVersion.VERSION_1_8 } } ``` --- # Treballar amb vistes - findViewById(R.id.some_id) - classes de les views: - TextView - Button ``` Button button = findViewById(R.id.rollBtn); ``` --- # TextView methods ``` view.setText("algun text"); view.setText(R.string.id_del_string); ``` --- # OnClick d'un botó ``` Button button; @Override protected void onCreate(Bundle savedInstanceState) { // (...) button = findViewById(R.id.buttonId); button.setOnClickListener(this::doSomething); } private void doSomething(View view) { // Do something } ``` --- # Toast Mostra un missatge per pantalla ``` Toast.makeText(this, "HELLO", Toast.LENGTH_LONG).show(); Toast.makeText(this, R.string.string_id, Toast.LENGTH_LONG).show(); ``` --- # Exercici - Al fer clic al botó mostra un toast dient que s'ha clicat al botó.
- Al fer clic al botó, modifica el text per "Dice rolled"
- Al fer clic mostra un número aleatori del 1 al 6
```java int randomInt = Random().nextInt(6) + 1; String randomIntString = randomInt + ""; ``` --- # Test automàtics - Redueix riscos - Reducció del temps de realitzar les proves - Executar tests de forma automàtica permet trobar errors que apareixen al tocar codi existent. --- # Test automàtics Cost vs benefíci. --- # Test projecte Android - Test - AndroidTest --- # AndroidTest - Espresso Dependencies Gradle ```groovy androidTestImplementation 'androidx.test:runner:1.2.0' androidTestImplementation 'androidx.test:rules:1.2.0' androidTestImplementation 'androidx.test.ext:junit:1.1.1' androidTestImplementation 'androidx.test.espresso:espresso-core:3.2.0' ``` --- # AndroidTest - Espresso - Indicar inici d'activitat en un test ``` @Rule public ActivityTestRule
mActivityTestRule = new ActivityTestRule<>(MainActivity.class); ``` --- # AndroidTest - Recordatori JUnit ``` @Test public void iWantSomethingToWorkTest() { // test code here } ``` --- # Androit Test - Espresso - ViewMatchers - Cercar una View - ViewActions - Acció a executar a la View - ViewAssertions - Comprovació que s'ha de complir (assert) a la View --- # AndroidTest - Espresso ``` // withId(R.id.my_view) is a ViewMatcher // click() is a ViewAction // matches(isDisplayed()) is a ViewAssertion onView(withId(R.id.my_view)) .perform(click()) .check(matches(isDisplayed())); ``` --- # AndroidTest - Espresso - ViewAssertions Algunes view assertions ``` matches(withText("someText")); // Té el text someText matches(withText("")) // No té text matches(not(withText(""))); // Té algun text ``` --- # Exercici - Fes el test: _startsWithNoDiceResultTest_
- Fes el test: _clickRollDisplaysResultTest_
--- # Seguent objectiu .fullscreen70[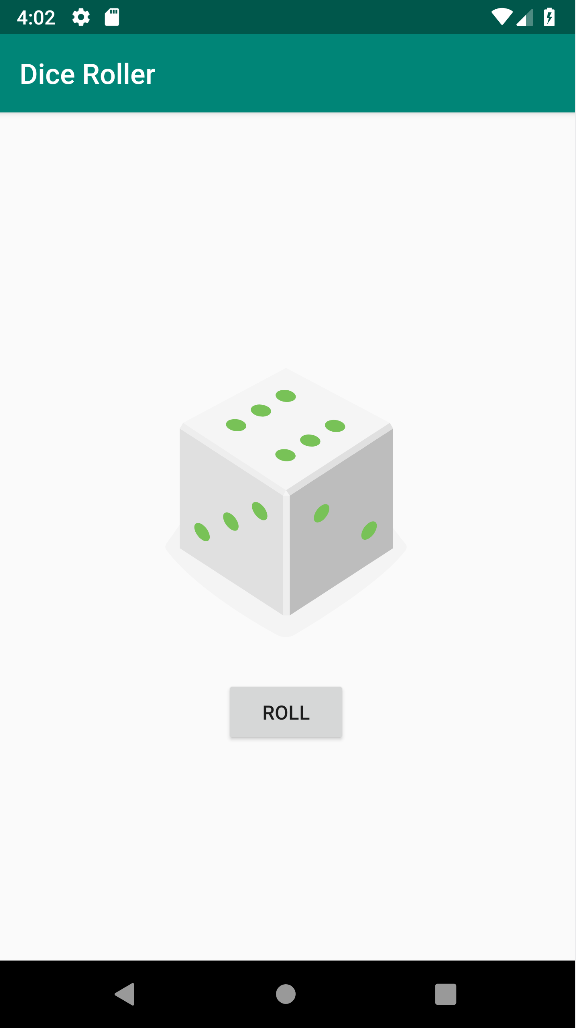] --- # Image resources - Diferents dispositius tenen diferents densitat (PPI) de pantalla - Nombre de píxels per polzada. - Android defineix els següents tipus: - mdpi - hdpi - xhdpi - xxhdpi - xxxhdpi --- # Image resources Com definim imatges per les diferents resolucions - Una imatge diferent per cada densitat - Usar un grafic vectorial (xml) --- # ImageView - Mostra una imatge - Algunes propietats d'un ImageView - src (xml) - android/tools - setImageResource (java) --- # Recursos [DiceImages.zip](exercicis/diceroller/DiceImages.zip) --- # Exercicis - Mostra l'imatge amb un dau segons el numero que hagi tocat. Ajuda: ``` private int getImageIdForNumber(int randomInt) { switch (randomInt){ case 1: return R.drawable.dice_1; case 2: return R.drawable.dice_2; case 3: return R.drawable.dice_3; case 4: return R.drawable.dice_4; case 5: return R.drawable.dice_5; default: return R.drawable.dice_6; } } ```
--- # Exercicis - Modifica els testos. - Afegeix les següents classes Java que et premetran fer ``` matches(withDrawable(R.drawable.some_drawable)) ``` [DrawableMatcher.java](exercicis/diceroller/DrawableMatcher.java) [EspressoTestsMatchers.java](exercicis/diceroller/EspressoTestsMatchers.java)
--- # Android API levels - compileSdkVersion - targetSdkVersion - minSdkVersion --- # Android API levels ## compileSdkVersion This parameter specifies the Android API level that Gradle should use to compile your app. This is the newest version of Android your app can support. That is, your app can use the API features included in this API level and lower. In this case your app supports API 28, which corresponds to Android 9 (Pie). --- # Android API levels ## targetSdkVersion This value is the most recent API that you have tested your app against. In many cases this is the same value as compileSdkVersion. --- # Android API levels ## minSdkVersion Oldest version of Android on which your app will run. Devices that run the Android OS older than this API level cannot run your app at all. https://developer.android.com/about/dashboards/ --- # Android appcompat - Permet usar funcionalitats noves en Apis més antigues - Activada per defecte ``` implementation 'androidx.appcompat:appcompat:1.1.0' ``` --- # Vector Drawables - compat - Compativilitat per defecte genera una imatge per cada resolució - Podem activar la compativilitat pels vector drawables A gradle, dins de defaultConfig ```groovy vectorDrawables.useSupportLibrary = true ``` Al layout ```xml app:srcCompat="@drawable/empty_dice" ```